```html
Smart Contract Code for Blockchain Forensics
Blockchain technology has revolutionized many industries, including forensics and investigations. Smart contracts,
with their selfexecuting nature and tamperproof characteristics, are increasingly utilized for various
forensic purposes. Below is a sample smart contract code tailored for blockchain forensics:
pragma solidity ^0.8.0;
contract ForensicContract {
// Define variables to store forensic data
address public investigator;
string public caseDescription;
uint256 public evidenceCount;
mapping(uint256 => string) public evidenceHashes;
// Event to log evidence submission
event EvidenceSubmitted(uint256 indexed evidenceId, string evidenceHash, string description, address sender);
// Modifier to restrict access to the investigator only
modifier onlyInvestigator() {
require(msg.sender == investigator, "Access restricted to investigator");
_;
}
// Constructor to initialize the contract
constructor(string memory _caseDescription) {
investigator = msg.sender;
caseDescription = _caseDescription;
evidenceCount = 0;
}
// Function to submit new evidence
function submitEvidence(string memory _evidenceHash, string memory _description) public onlyInvestigator {
evidenceCount ;
evidenceHashes[evidenceCount] = _evidenceHash;
emit EvidenceSubmitted(evidenceCount, _evidenceHash, _description, msg.sender);
}
// Function to retrieve evidence hash by evidence ID
function getEvidenceHash(uint256 _evidenceId) public view returns (string memory) {
require(_evidenceId > 0 && _evidenceId <= evidenceCount, "Invalid evidence ID");
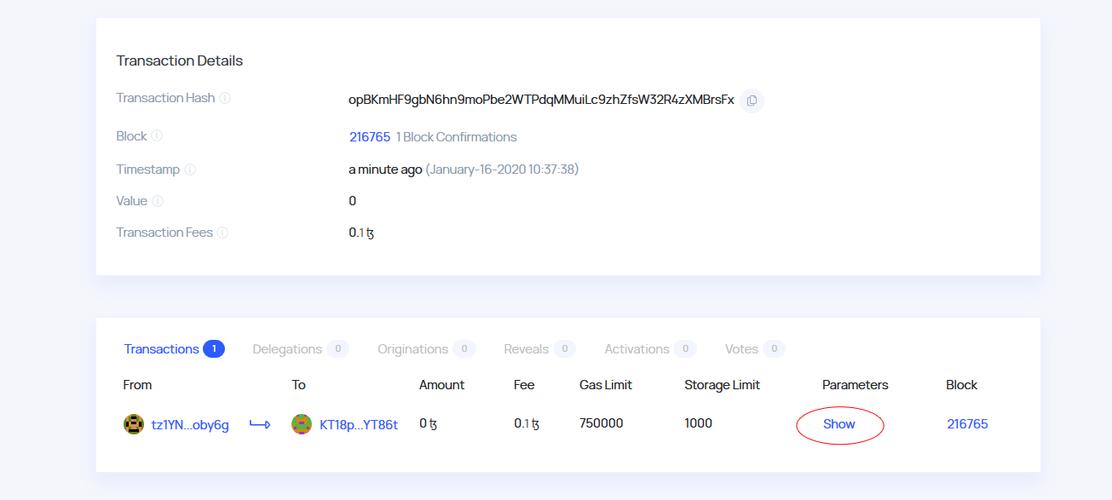
return evidenceHashes[_evidenceId];
}
}
This smart contract serves as a foundation for managing forensic data on the blockchain. Let's break down its
key components:
- Variables: The contract stores the address of the investigator, case description, evidence
count, and evidence hashes.
- Events: An event is triggered upon submitting new evidence, logging essential information
such as evidence ID, hash, description, and sender address.
- Modifier: The
onlyInvestigator
modifier ensures that only the designatedinvestigator can access certain functions.
- Constructor: Initializes the contract with the investigator's address and case description.
- Functions:
submitEvidence
: Allows the investigator to submit new evidence along with its hash anddescription.
getEvidenceHash
: Enables anyone to retrieve the evidence hash by providing the evidenceID.
By deploying this smart contract on a blockchain network, forensic investigators can securely manage and
timestamp evidence, ensuring its integrity and traceability throughout the investigation process.